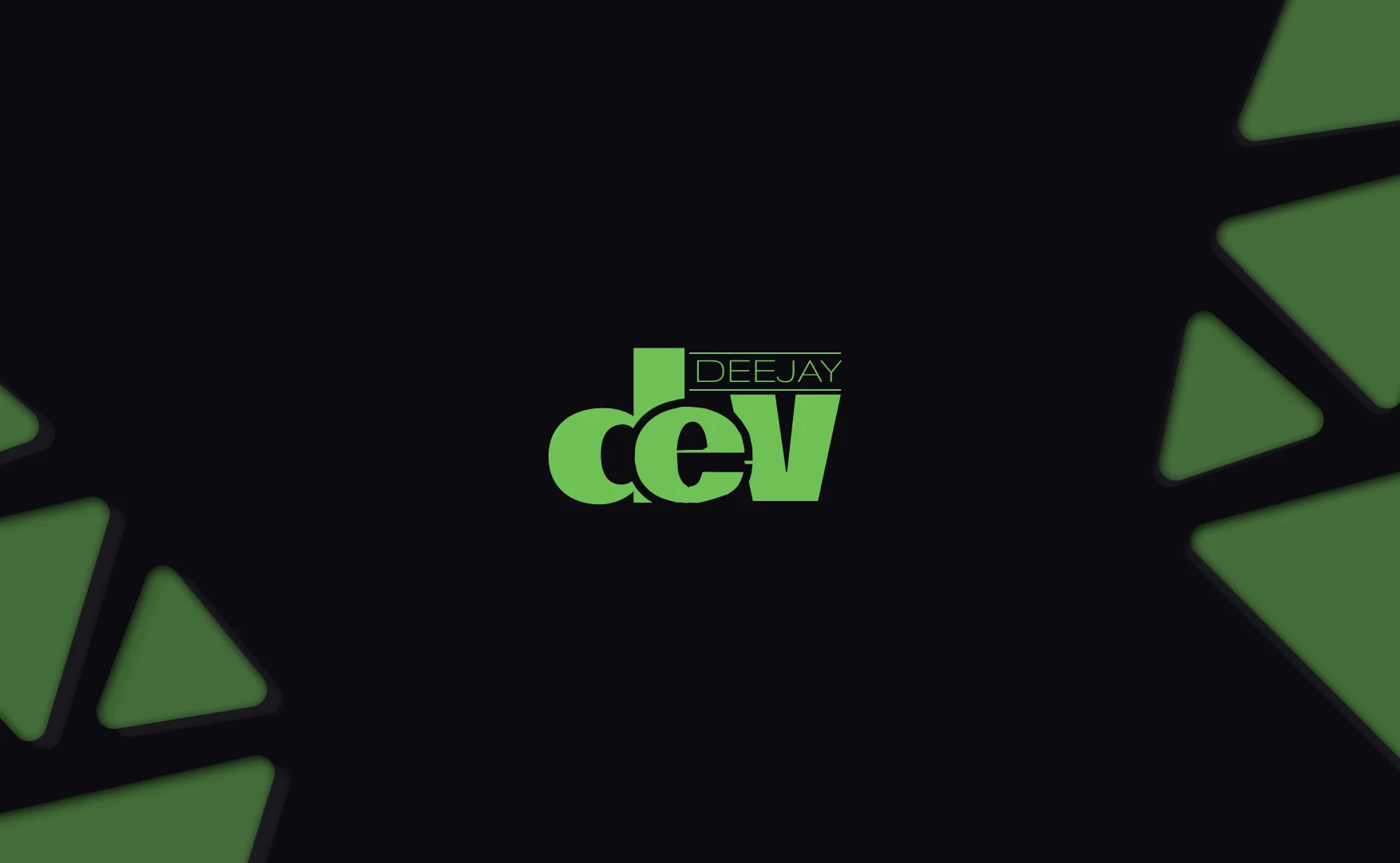
Crazy JS Concepts to Know SOLD OUT
$5 $9.99
Last updated: 6/2024
Description
This ebook is a comprehensive guide covering essential JavaScript concepts that every developer should know. The following list presents the topics in a new order to enhance the flow of information and learning experience:
- Clean Code
- Design Patterns
- Data Structures
- Algorithms
- Collections and Generators
- Inheritance, Polymorphism, Code Reusability
- Error Handling (try…catch)
- ES6 Modules
- WebSockets, Socket.IO
- Fetch API and AJAX
- LocalStorage, SessionStorage
- Promises
- async/await
- High Order Functions
- Closures
- Recursion
- Pure Functions, Side Effects, State Mutation, Event Propagation
- Function Scope, Block Scope, Lexical Scope
- Object.create, Object.assign
- Prototypal Inheritance, Prototype Chain
- Factories, Classes
- new, Constructor, instanceof, Instances
- DOM, Document Trees
- IIFE, Modules, Namespaces
- Bitwise Operators, Typed Arrays, Array Buffers
- JavaScript Engines
- Message Queue, Event Loop
- setTimeout, setInterval, requestAnimationFrame
- Call Stack
- Primitive Types
- Value Types, Reference Types
- Implicit, Explicit, Nominal, Structural, Duck Typing
- == vs === vs typeof
- Expression vs Statement
- map, reduce, filter
- Ternary Operator
- Spread and Rest Operators
- Destructuring
- Template Literals
- Arrow Functions
- Array Methods (forEach, some, every, find, findIndex)
- String Methods (split, trim, replace)
- Object Methods (keys, values, entries)
- Math Methods (floor, ceil, random)
- JSON and Object Serialization/Deserialization
- Costly Operations, Big O Notation
- Partial Application, Currying, Composition, Pipe
- Implicit vs Explicit Coercion
- Event Bubbling vs Capturing
- Testing with Jest or Mocha
Whether you are a beginner or an experienced developer, this book aims to enhance your understanding of JavaScript and improve your coding skills.